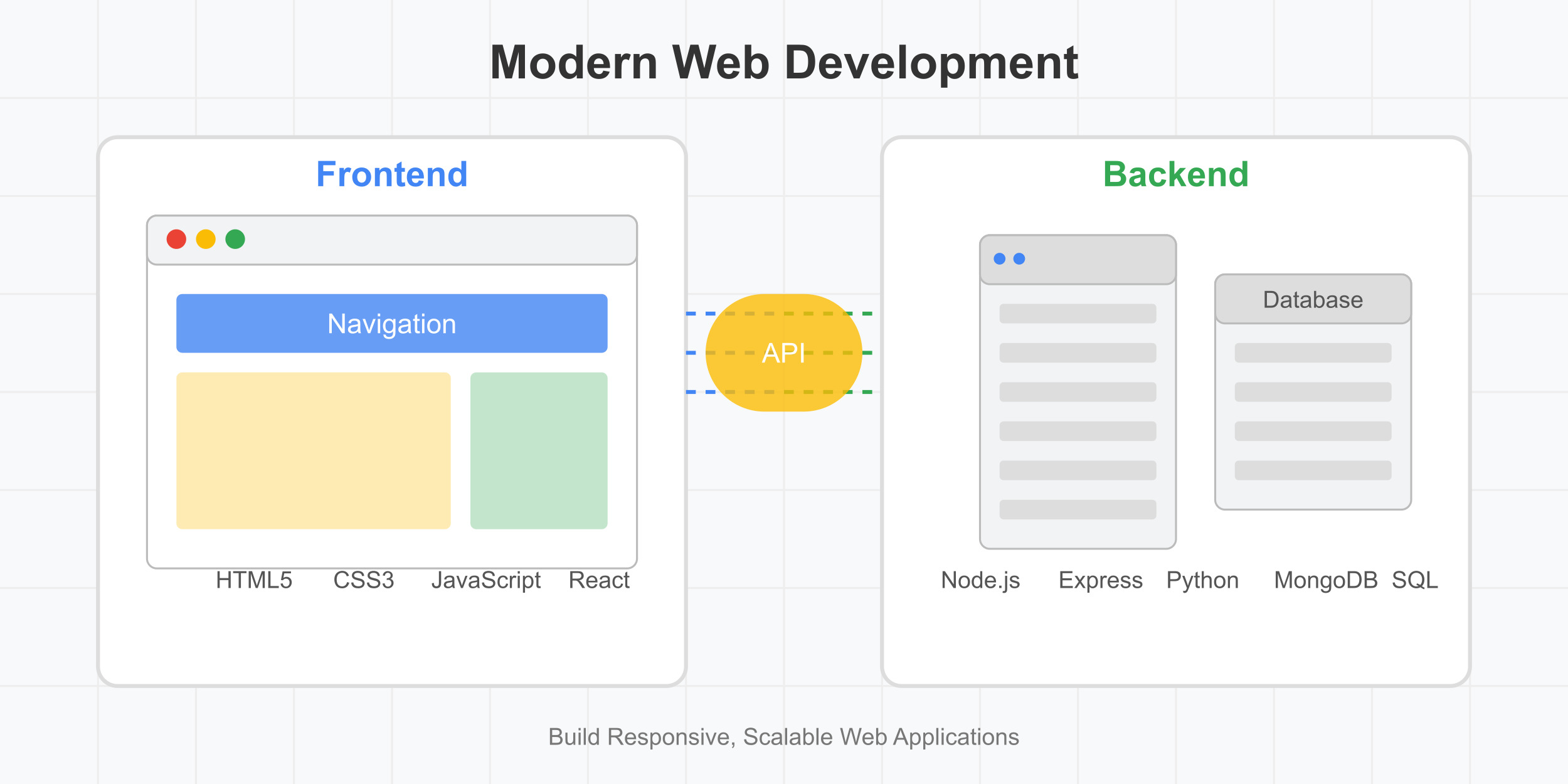
Introduction
Cybersecurity is an essential aspect of software development, yet many university students overlook security best practices when writing code for their projects. Whether you’re developing a small web application or a large-scale software project, writing secure code is crucial to prevent vulnerabilities that could be exploited. In the U.S., universities emphasize secure coding principles, aligning with guidelines from organizations such as the National Institute of Standards and Technology (NIST) and the Open Web Application Security Project (OWASP).
This article explores fundamental cybersecurity principles for students, highlighting best practices to help them write secure code in university projects.
Understanding Common Security Vulnerabilities
Before diving into secure coding techniques, it’s essential to understand the most common vulnerabilities that plague software applications. Here are a few key threats:
1. SQL Injection
SQL injection occurs when an attacker manipulates a database query by inserting malicious SQL statements. This can lead to unauthorized access, data leaks, or even complete database destruction.
Prevention: Use parameterized queries and prepared statements in SQL to prevent injection attacks. In Python, for example:
cursor.execute("SELECT * FROM users WHERE username = ?", (username,))
2. Cross-Site Scripting (XSS)
XSS attacks allow attackers to inject malicious scripts into web applications, potentially compromising user data or stealing session cookies.
Prevention: Always escape user inputs, use Content Security Policy (CSP), and sanitize data before rendering it in a browser.
3. Cross-Site Request Forgery (CSRF)
CSRF attacks trick users into executing unwanted actions on a web application where they are authenticated.
Prevention: Implement CSRF tokens to verify the authenticity of user requests.
4. Insecure Authentication and Authorization
Improper authentication mechanisms can allow attackers to gain unauthorized access to sensitive data.
Prevention: Use multi-factor authentication (MFA), secure password storage (bcrypt or Argon2), and enforce strong password policies.
Best Practices for Writing Secure Code
With a clear understanding of security threats, students should implement the following secure coding practices:
1. Validate and Sanitize User Input
Never trust user input. Always validate input against a strict set of rules and sanitize it to remove malicious content.
2. Use Secure Coding Frameworks
Many modern frameworks offer built-in security features. In the U.S., universities often recommend Django for Python or Spring Security for Java to enforce security best practices.
3. Secure API Endpoints
If your project involves APIs, ensure they are secured by:
- Implementing proper authentication (OAuth 2.0, JWTs)
- Using HTTPS to encrypt data in transit
- Validating all incoming requests
4. Keep Dependencies Updated
Outdated libraries and frameworks are common entry points for attackers. Use tools like Dependabot or Snyk to monitor and update dependencies regularly.
5. Implement Proper Error Handling
Generic error messages can expose system details to attackers. Instead, log detailed errors on the server while displaying user-friendly messages.
University Cybersecurity Initiatives
Many U.S. universities incorporate cybersecurity into their curriculum. Schools like MIT, Stanford, and the University of California, Berkeley, offer dedicated courses on secure coding. Additionally, programs like the National Cybersecurity Alliance promote awareness and education among students.
Some universities also participate in Capture The Flag (CTF) competitions, where students test their cybersecurity skills in a controlled environment. If your university offers such opportunities, participating can help you apply security concepts practically.
Tools for Writing Secure Code
Several tools can help students improve security in their projects:
- Static Analysis Tools: SonarQube, Bandit (for Python), ESLint (for JavaScript)
- Dependency Checkers: OWASP Dependency-Check, Retire.js
- Penetration Testing Tools: Burp Suite, OWASP ZAP
Using these tools during development can identify vulnerabilities before deployment.
Conclusion
Writing secure code is a responsibility that extends beyond the classroom. By understanding common vulnerabilities, following secure coding practices, and utilizing available tools, students can build more resilient software. As cybersecurity threats continue to evolve, staying informed and applying best practices will help future developers contribute to a safer digital world.
Author Bio: Emily is a programming tutor and technical writer specializing in secure coding and software development. She collaborates with subject-matter experts to create in-depth content that helps students improve their coding practices. Emily also provides programming homework help, guiding students through complex topics to ensure they write robust, secure code.
Also Read: Secrets to Balanced University Life